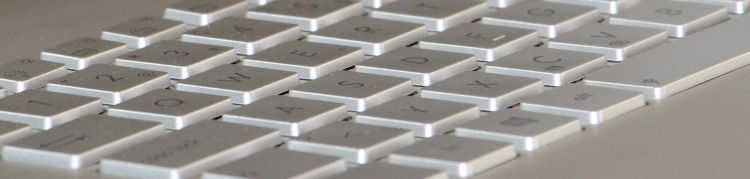
In this article, we saw how to use a GPU program with GLSL Hacker. Now we’re going to see how we can pass information (variables) to a GPU program.
GLSL Hacker does not re-invent the wheel. In OpenGL, shader input variables are called uniform variables or uniforms in short. OpenGL has a set of functions to deal with uniform variables: glUniform1f(), glUniform4f() and so on. GLSL Hacker uses the same way and syntax to pass data to a shader. All functions that pass values to a shader are in the gh_gpu_program lib:
gh_gpu_program.uniform1f() gh_gpu_program.uniform4f() ... gh_gpu_program.uniform1i() ...
OK let’s see a simple example. We have a shader that performs texturing and we want to modulate the final fragment color with a custom color. The fragment shader has two uniforms: the texture sampler (tex0 on texture unit 0) and the custom color (color):
... gh_gpu_program.bind(gpu_prog) gh_gpu_program.uniform1i(gpu_prog, "tex0", 0) gh_gpu_program.uniform4f(gpu_prog, "color", 1.0, 0.0, 0.5, 1.0) gh_object.render(mesh) ...
And here is the code of this pixel shader:
#version 150 in vec4 Vertex_UV; // From the vertex shader uniform sampler2D tex0; uniform vec4 color; out vec4 FragColor; // Fragment shader output. void main (void) { vec2 uv = Vertex_UV.xy; uv.y *= -1.0; vec3 t = texture(tex0,uv).rgb; FragColor = vec4(t * color.rgb, 1.0); }