The new gfx03.lua helper lib, available with GeeXLab 0.9.4.0+ (in GeeXLab/libs/lua/) is an improved version of gfx02.lua.
The goal of this helper lib is to provide a simple framework to quickly code a demo: fonts with FTGL are automatically loaded, a background quad with a blue-metal gradient is ready to use. Some basic GPU programs (color, vertex color, texture, phong) are available for all platforms including Raspberry Pi. Information such as elapsed time, FPS or renderer model is displayed as well. So if you need to quickly test a GPU program, you can copy and paste this minimal demo, update the GPU program and Lua code accordingly.
Here is a code that shows a minimal use of gfx03.lua. The demo is available in the code sample pack (host_api/gfx03_minimal.xml). The payload (your own code) is a quad rendered with a simple shader.
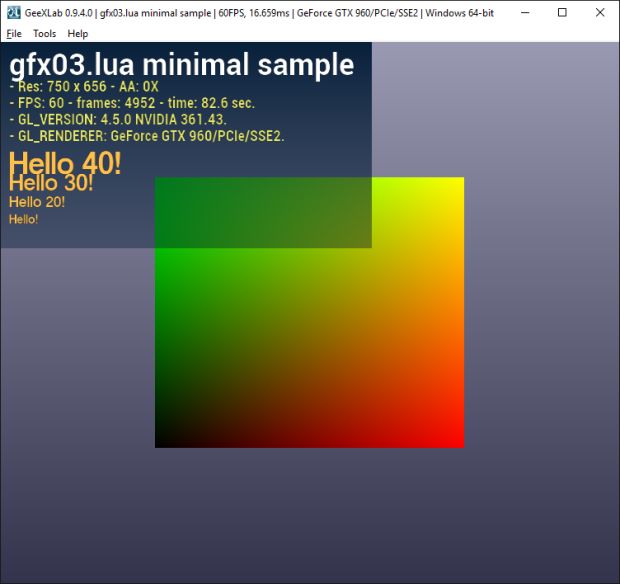
INIT script
--[[ Load and init the GFX v3 helper library. --]] local lib_dir = gh_utils.get_scripting_libs_dir() dofile(lib_dir .. "lua/gfx03.lua") gfx.init() gfx.vsync(1) gfx.display_common_info(1) gfx.show_info_quad(1) -- for better visibility gfx.update_info_quad_size(450, 250) -- new size for the info quad if (gfx.is_rpi() == 1) then gfx.mouse.show(1) end gfx.set_main_title("gfx03.lua minimal sample") winW, winH = gfx.window.getsize() -- Your code here -------------- camera_ortho = gfx.camera.create_ortho(0, 0, winW, winH) gpu_prog = gh_node.getid("gpu_prog") -- Your code here --------------
TERMINATE script
gfx.terminate()
FRAME script
gfx.begin_frame() local elapsed_time = gfx.get_time() local dt = gfx.get_dt() local mx, my = gfx.mouse.get_position() gfx.draw_bkg_quad() -- Your code here -------------- gh_camera.bind(camera_ortho) gh_renderer.set_depth_test_state(0) gh_gpu_program.bind(gpu_prog) gh_gpu_program.uniform1f(gpu_prog, "u_time", elapsed_time) gfx.draw_quad(0, 0, 0.5*winW, 0.5*winH) -- Your code here -------------- gfx.write_text_40(10, 160, 1.0, 0.75, 0.3, 1, "Hello 40!") gfx.write_text_30(10, 180, 1.0, 0.75, 0.3, 1, "Hello 30!") gfx.write_text_20(10, 200, 1.0, 0.75, 0.3, 1, "Hello 20!") gfx.write_text(10, 220, 1.0, 0.75, 0.3, 1, "Hello!") gfx.end_frame(1)
SIZE script
winW, winH = gfx.window.getsize() gfx.resize(winW, winH) -- Your code here -------------- gfx.camera.update_ortho(camera_ortho, 0, 0, winW, winH) -- Your code here --------------
The GPU program is really basic:
If you only want to display an image, you can load the texture with gfx.texture.load and use one of the available GPU programs: “texture” in that case. All GPU programs are available in shaderlib.lua (“color”, “vertex color”, “texture”, “phong”, “phong_texture”).
INIT script
... local demo_dir = gh_utils.get_demo_dir() tex = gfx.texture.load(demo_dir .. "data/tarte_fruits.jpg", "rgba_u8") texture_prog = gfx.gpu_program.getid("texture")
FRAME script
... gh_camera.bind(camera_ortho) gh_renderer.set_depth_test_state(0) gh_texture.bind(tex, 0) gh_gpu_program.bind(texture_prog) gfx.draw_quad(0, 0, 0.8*winW, 0.8*winH) ...
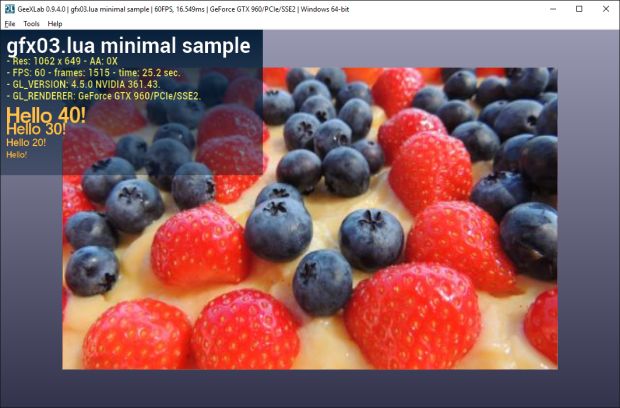
As soon as I find a new function that can save coding time, I will update gfx03.lua.