If you need to retrieve date and time in Lua, here is small demo that displays the current date as well as elapsed time in seconds.
Lua has two functions for time queries from the os lib: os.time() and os.date().
os.time() returns the timestamp, which is the number of second since beginning of time (more or less January 1, 1970).
os.date() returns a structure that contains the year, month, day, hour, minute and second of a particular timestamp.
The GeeXLab demo is available in host_api/Lua_Mics/date_time/ folder of the code sample pack.
Code snippet:
local timestamp = os.time() local tmp = os.date("*t", timestamp) local date_str = string.format("year: %d, month: %d, day: %d, hour: %d, min: %d, sec: %d", tmp.year, tmp.month, tmp.day, tmp.hour, tmp.min, tmp.sec)) gh_utils.font_render(font, 10, 20, 1.0, 1.0, 1.0, 1.0, date_str) local day_seconds = tmp.hour * 3600 + tmp.min * 60 + tmp.sec gh_utils.font_render(font, 10, 40, 0.2, 1.0, 0.0, 1.0, "Elapsed time:") gh_utils.font_render(font, 20, 60, 0.9, 1.0, 0.0, 1.0, string.format("- since demo start: %.1f sec", elapsed_time)) gh_utils.font_render(font, 20, 80, 0.9, 1.0, 0.0, 1.0, string.format("- since day start: %.1f sec", day_seconds))
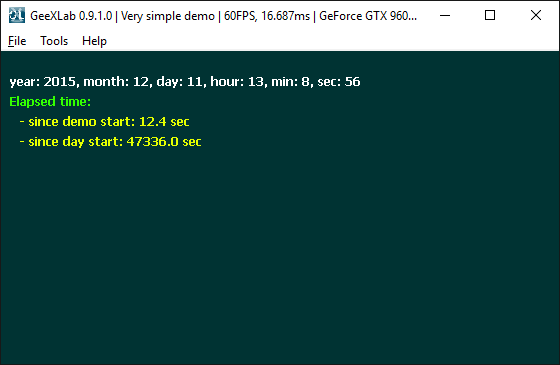
In some Shadertoy demos there is an uniform variable called: iDate which is a vec4:
uniform vec4 iDate;
You can update this uniform in a FRAME script with the following code:
... local timestamp = os.time() local tmp = os.date("*t", timestamp) local time_seconds = tmp.hour * 3600 + tmp.min * 60 + tmp.sec gh_gpu_program.uniform4f(shadertoy_prog, "iDate", tmp.year, tmp.month, tmp.day, time_seconds) ...
References